UPDATE 2022: The MAX7219LED8x8 library, now renamed to MAX7219tiny has now a new home at tinusaur.com/libraries/max7219tiny. Check also this MAX7219 & ATtiny85 tutorial to learn how the library works.
The MAX7219 controller manufactured by Maxim Integrated is a compact, serial input/output common-cathode display driver that could interface microcontrollers to 64 individual LEDs, 7-segment numeric LED displays of up to 8 digits, bar-graph displays, etc. Included on-chip are a BCD code-B decoder, multiplex scan circuitry, segment and digit drivers, and an 8×8 static RAM that stores each digit.
The MAX7219 modules are very convenient to use with microcontrollers such as ATtiny85, or, in our case the Tinusaur Board.
The Hardware
The MAX7219 modules usually look like this:
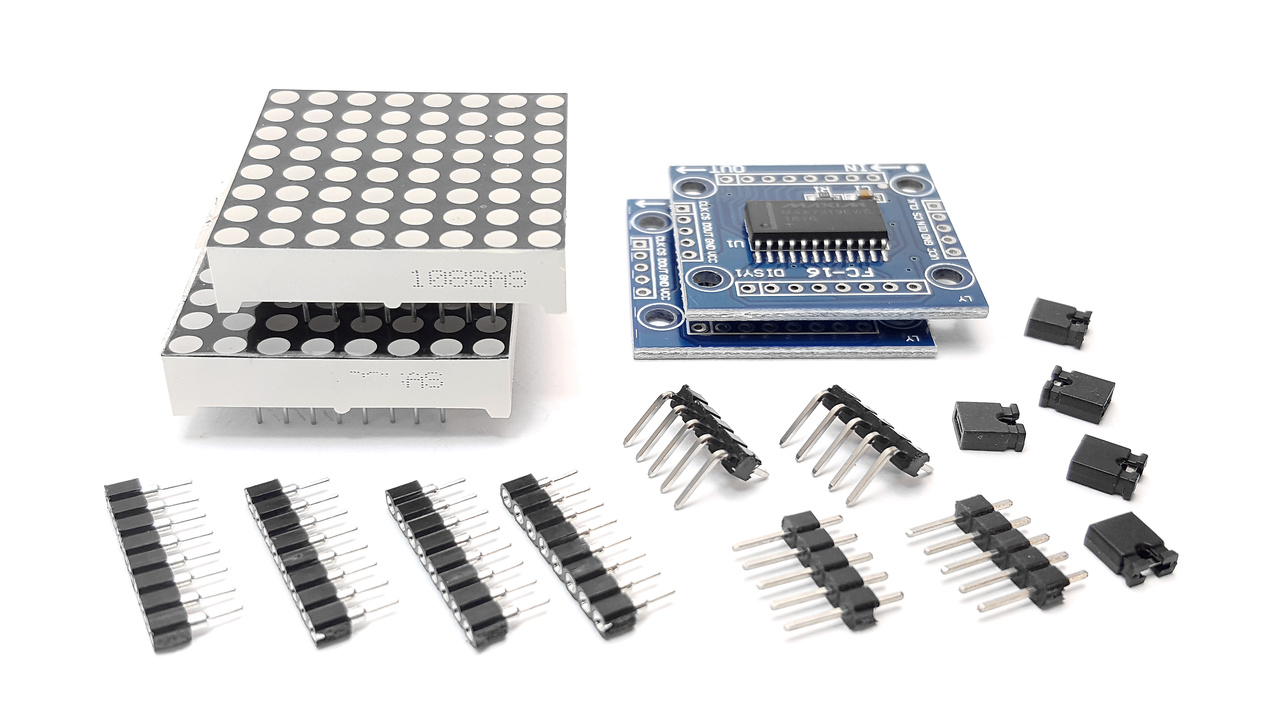
They have an input bus on one side and output bus on the other. This allows you to daisy chain 2 or more modules, i.e. one after another, to create more complicated setups.
The modules that we are using are capable of connecting in a chain using 5 small jumpers. See the picture below.
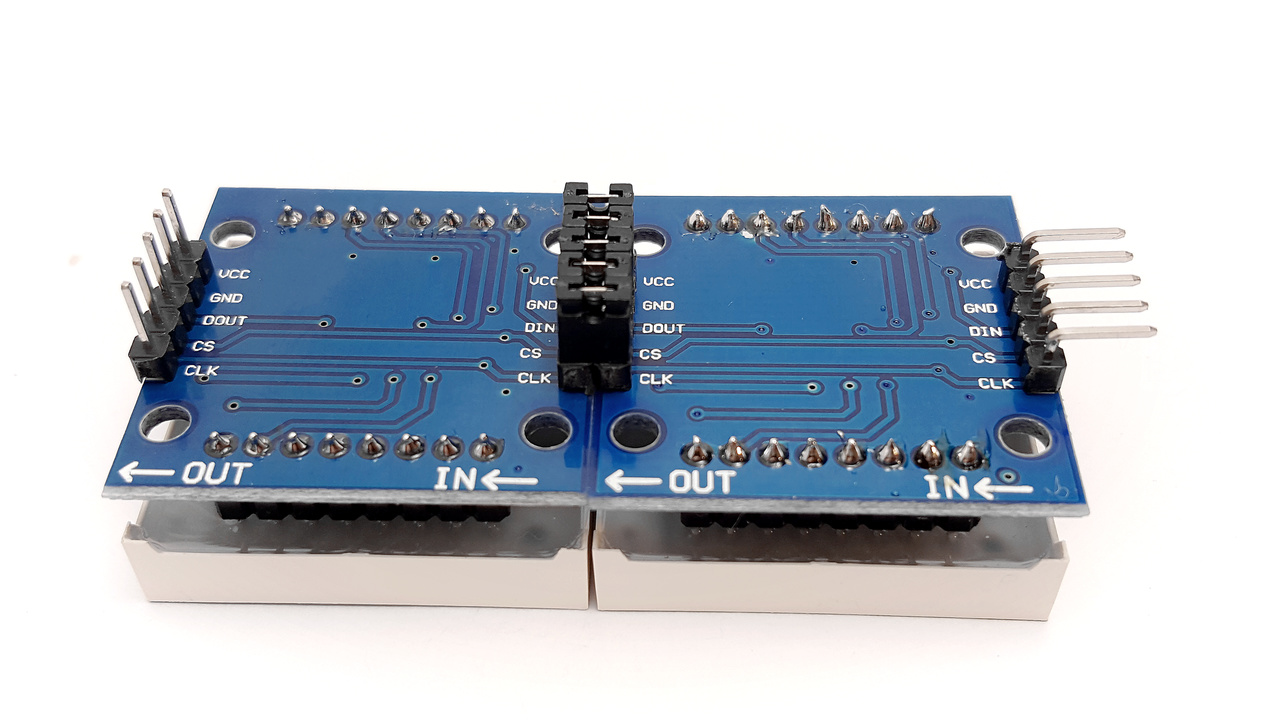
Pinout and Signals
MAX7219 module has 5 pins:
- VCC – power (+)
- GND – ground (-)
- DIN – Data input
- CS – Chip select
- CLK – Clock
That means that we need 3 pins on the ATtiny85 microcontroller side to control the module. Those will be:
- PB0 – connected to the CLK
- PB1 – connected to the CS
- PB2 – connected to the DIN
This is sufficient to connect to the MAX7219 module and program it.
The Protocol
Communicating with the MAX7219 is relatively easy – it uses a synchronous protocol which means that for every data bit we send there is a clock cycle that signifies the presence of that data bit.

In other words, we send 2 parallel sequences to bits – one for the clock and another for the data. This is what the software does.
The Software
The way this MAX7219 module works is this:
- We write bytes to its internal register.
- MAX7219 interprets the data.
- MAX7219 controls the LEDs in the matrix.
That also means that we don’t have to circle through the array of LEDs all the time in order to light them up – the MAX7219 controller takes care of that. It could also manage the intensity of the LEDs.
So, to use the MAX7219 modules in a convenient way we need a library of functions to serve that purpose.
First, we need some basic functions in order to write to the MAX7219 registers.
- Writing a byte to the MAX7219.
- Writing a word (2 bytes) to the MAX7219.
The function that writes one byte to the controller looks like this:
void max7219_byte(uint8_t data) {
for(uint8_t i = 8; i >= 1; i--) {
PORTB &= ~(1 << MAX7219_CLK); // Set CLK to LOW
if (data & 0x80) // Mask the MSB of the data
PORTB |= (1 << MAX7219_DIN); // Set DIN to HIGH
else
PORTB &= ~(1 << MAX7219_DIN); // Set DIN to LOW
PORTB |= (1 << MAX7219_CLK); // Set CLK to HIGH
data <<= 1; // Shift to the left
}
}
Now that we can send bytes to the MAX7219 we can start sending commands. This is done by sending 2 byes – 1st for the address of the internal register and the 2nd for the data we’d like to send.
There is more than a dozen of register in the MAX7219 controller.
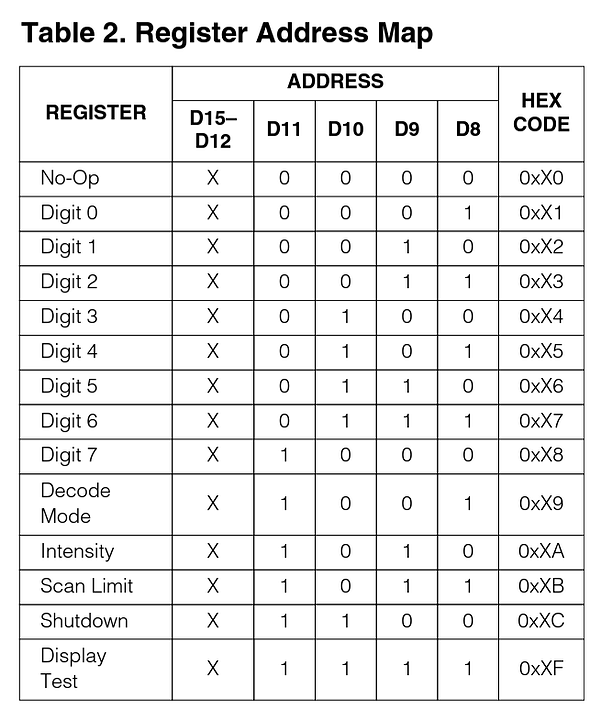
Sending a command, or a word, is basically sending 2 consecutive bytes. The function implementing that is very simple.
void max7219_word(uint8_t address, uint8_t data) {
PORTB &= ~(1 << MAX7219_CS); // Set CS to LOW
max7219_byte(address); // Sending the address
max7219_byte(data); // Sending the data
PORTB |= (1 << MAX7219_CS); // Set CS to HIGH
PORTB &= ~(1 << MAX7219_CLK); // Set CLK to LOW
}
It is important to note here the line where we bring the CS signal back to HIGH – this marks the end of the sequence – in this case, the end of the command. A similar technique is used when controlling more than one matrix connected in a chain.
Next step, before we start turning on and off the LEDs, is to initialize the MAX7219 controller. This is done by writing certain values to certain registers. For convenience, while coding it we could put the initialization sequence in an array.
uint8_t initseq[] = {
0x09, 0x00, // Decode-Mode Register, 00 = No decode
0x0a, 0x01, // Intensity Register, 0x00 .. 0x0f
0x0b, 0x07, // Scan-Limit Register, 0x07 to show all lines
0x0c, 0x01, // Shutdown Register, 0x01 = Normal Operation
0x0f, 0x00, // Display-Test Register, 0x00 = Normal Operation
};
We just need to send the 5 commands above in a sequence as address/data pairs.
Next step – lighting up a row of LEDs.
This is very simple – we just write one command where 1st byte is the address (from 1 to 8) and the 2nd byte is the 8 bits representing the 8 LEDs in the row.
void max7219_row(uint8_t address, uint8_t data) {
if (address >= 1 && address <= 8) max7219_word(address, data);
}
It is important to note that this will work for 1 matrix only. If we connect more matrices in a chain they will all show the same data. The reason for this is that after sending the command we bring the CS signal back to HIGH which causes all the MAX7219 controllers in the chain to latch and show whatever the last command was.
Testing
This is a simple testing program that lights up a LED on the first row (r=1) on the right-most position, then moves that on the left until it reaches the left-most position, then does the same on one row up (r=2) )until it reaches the top (r=8).
max7219_init();
for (;;) {
for (uint8_t r = 1; r <= 8; r++) {
uint8_t d = 1;
for (uint8_t i = 9; i > 0; i--) {
max7219_row(r, d);
d = d << 1;
_delay_ms(50);
}
}
}
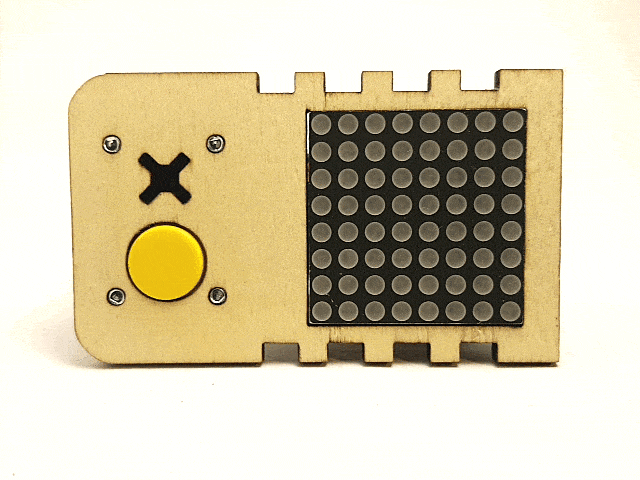
This testing code doesn’t do much but it demonstrates how to communicate with the MAX7219 controller.
The MAX7219LED8x8 Library
All of the functions mentioned above are part of the MAX7219LED8x8 library. Its source code is available at https://bitbucket.org/tinusaur/max7219led8x8.
The Tinusaur Shield GAMEx3
If you already have a Tinusaur Board we have the Shield GAMEx3 for it to connect a MAX7219 module easier to your ATtiny85 microcontroller.
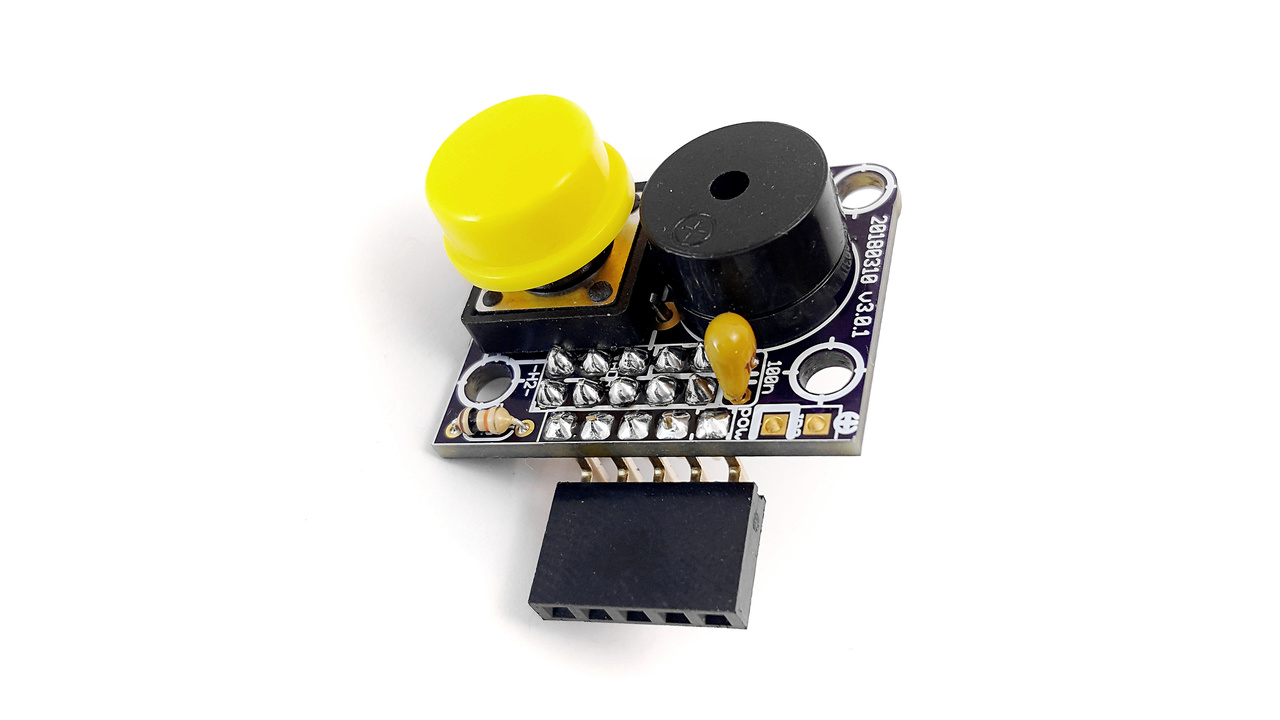
The Gametinu Project
The Gametinu is a small game platform that you could build yourself using the Shield GAMEx3 and a few more parts and tools.
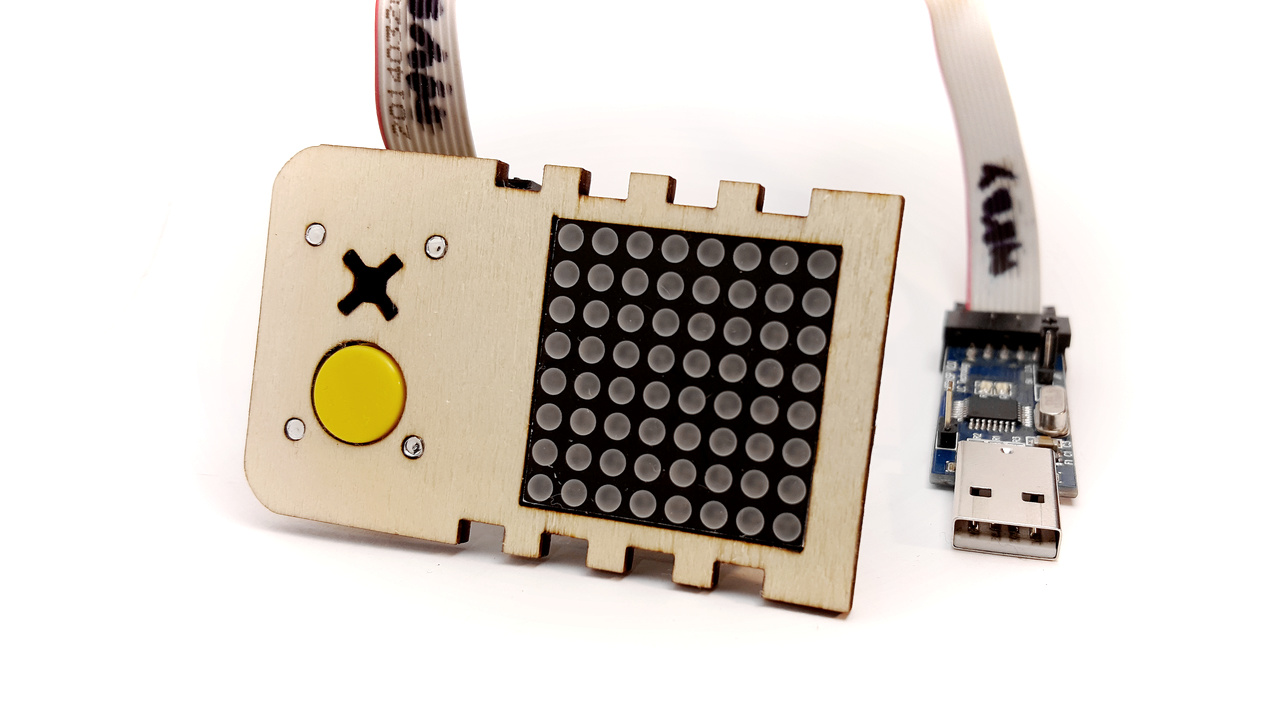
References
MAX7219 specification and datasheet:
- http://www.maximintegrated.com/…/MAX7219.html
- http://datasheets.maximintegrated.com/en/ds/MAX7219-MAX7221.pdf
This article is a rewritten version of another article from 2014:
MAX7219 driver for LED Matrix 8×8.